ตัวอย่าง EA แบบ Grid Trading
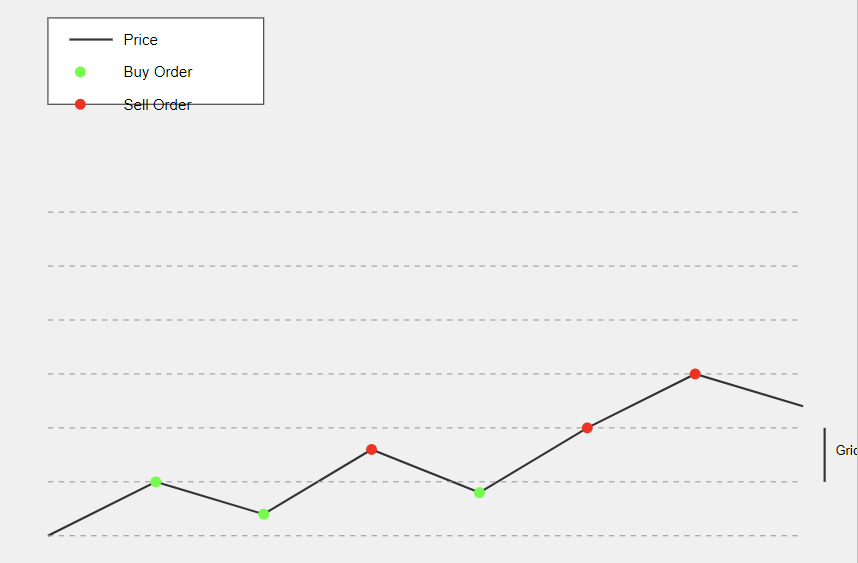
คำอธิบายองค์ประกอบหลักของ EA แบบ Grid Trading:
- Input Parameters:
- GridSize: ระยะห่างระหว่างแต่ละระดับของ grid (ในหน่วย pips)
- LotSize: ขนาดล็อตสำหรับแต่ละออเดอร์
- MaxLevels: จำนวนระดับสูงสุดของ grid
- TakeProfit: ระยะ Take Profit สำหรับแต่ละออเดอร์ (ในหน่วย pips)
- StopLoss: ระยะ Stop Loss สำหรับออเดอร์แรกของแต่ละทิศทาง (ในหน่วย pips)
- OnInit():
- ตรวจสอบความถูกต้องของ input parameters
- OnTick():
- ตรวจสอบและเปิดระดับ grid ใหม่ถ้าจำเป็น
- ตรวจสอบและปิด grid ทั้งหมดถ้าเงื่อนไขเป็นจริง
- CheckAndOpenGridLevels():
- ตรวจสอบว่าควรเปิดระดับ grid ใหม่หรือไม่ทั้งฝั่ง Buy และ Sell
- GetNextBuyLevel() และ GetNextSellLevel():
- คำนวณระดับราคาสำหรับออเดอร์ถัดไปในแต่ละทิศทาง
- OpenBuyOrder() และ OpenSellOrder():
- เปิดออเดอร์ Buy หรือ Sell ที่ระดับราคาที่กำหนด
- CheckAndCloseGrid():
- ตรวจสอบกำไรรวมของทั้ง grid และปิดทั้งหมดถ้าถึงเป้าหมาย
- CloseAllOrders():
- ปิดออเดอร์ทั้งหมดที่เกี่ยวข้องกับ grid
EA นี้จะสร้าง grid ของออเดอร์ทั้งฝั่ง Buy และ Sell โดยมีระยะห่างเท่ากันตาม GridSize ที่กำหนด เมื่อราคาเคลื่อนที่ไปถึงระดับใดๆ ของ grid EA จะเปิดออเดอร์ใหม่ที่ระดับนั้น จนกว่าจะถึงจำนวน MaxLevels EA จะปิดทั้ง grid เมื่อกำไรรวมถึงเป้าหมายที่กำหนด
//+------------------------------------------------------------------+
//| Grid Trading EA.mq4 |
//| Copyright 2024, MetaQuotes Software Corp. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2024, MetaQuotes Software Corp."
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
// Input parameters
input double GridSize = 20; // Grid size in pips
input double LotSize = 0.01; // Lot size for each grid level
input int MaxLevels = 10; // Maximum number of grid levels
input double TakeProfit = 20; // Take profit in pips
input double StopLoss = 200; // Stop loss in pips (from the first grid level)
input int MagicNumber = 12345; // Magic number for order identification
// Global variables
double g_pip;
int g_totalBuyOrders = 0;
int g_totalSellOrders = 0;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
// Initialize pip value
g_pip = Point * 10;
// Validate input parameters
if (GridSize <= 0 || LotSize <= 0 || MaxLevels <= 0 || TakeProfit <= 0 || StopLoss <= 0)
{
Print("Invalid input parameters. Please check and try again.");
return INIT_PARAMETERS_INCORRECT;
}
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
// Clean up any resources if needed
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
// Check if we need to open new grid levels
CheckAndOpenGridLevels();
// Check if we need to close the entire grid
CheckAndCloseGrid();
}
//+------------------------------------------------------------------+
//| Check and open new grid levels |
//+------------------------------------------------------------------+
void CheckAndOpenGridLevels()
{
double currentPrice = (Ask + Bid) / 2;
// Check buy grid
if (g_totalBuyOrders < MaxLevels)
{
double nextBuyLevel = GetNextBuyLevel();
if (currentPrice <= nextBuyLevel)
{
OpenBuyOrder(nextBuyLevel);
}
}
// Check sell grid
if (g_totalSellOrders < MaxLevels) { double nextSellLevel = GetNextSellLevel(); if (currentPrice >= nextSellLevel)
{
OpenSellOrder(nextSellLevel);
}
}
}
//+------------------------------------------------------------------+
//| Get the price level for the next buy order |
//+------------------------------------------------------------------+
double GetNextBuyLevel()
{
if (g_totalBuyOrders == 0)
{
return Ask;
}
else
{
double lowestBuyPrice = DBL_MAX;
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderMagicNumber() == MagicNumber && OrderType() == OP_BUY)
{
lowestBuyPrice = MathMin(lowestBuyPrice, OrderOpenPrice());
}
}
}
return lowestBuyPrice - GridSize * g_pip;
}
}
//+------------------------------------------------------------------+
//| Get the price level for the next sell order |
//+------------------------------------------------------------------+
double GetNextSellLevel()
{
if (g_totalSellOrders == 0)
{
return Bid;
}
else
{
double highestSellPrice = 0;
for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) { if (OrderMagicNumber() == MagicNumber && OrderType() == OP_SELL) { highestSellPrice = MathMax(highestSellPrice, OrderOpenPrice()); } } } return highestSellPrice + GridSize * g_pip; } } //+------------------------------------------------------------------+ //| Open a buy order | //+------------------------------------------------------------------+ void OpenBuyOrder(double price) { double sl = g_totalBuyOrders == 0 ? price - StopLoss * g_pip : 0; double tp = price + TakeProfit * g_pip; int ticket = OrderSend(Symbol(), OP_BUY, LotSize, price, 3, sl, tp, "Grid Buy", MagicNumber, 0, Blue); if (ticket > 0)
{
g_totalBuyOrders++;
Print("Buy order opened at ", price);
}
else
{
Print("Error opening buy order: ", GetLastError());
}
}
//+------------------------------------------------------------------+
//| Open a sell order |
//+------------------------------------------------------------------+
void OpenSellOrder(double price)
{
double sl = g_totalSellOrders == 0 ? price + StopLoss * g_pip : 0;
double tp = price - TakeProfit * g_pip;
int ticket = OrderSend(Symbol(), OP_SELL, LotSize, price, 3, sl, tp, "Grid Sell", MagicNumber, 0, Red);
if (ticket > 0)
{
g_totalSellOrders++;
Print("Sell order opened at ", price);
}
else
{
Print("Error opening sell order: ", GetLastError());
}
}
//+------------------------------------------------------------------+
//| Check and close the entire grid if necessary |
//+------------------------------------------------------------------+
void CheckAndCloseGrid()
{
if (g_totalBuyOrders > 0 && g_totalSellOrders > 0)
{
double totalProfit = 0;
for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES)) { if (OrderMagicNumber() == MagicNumber) { totalProfit += OrderProfit() + OrderSwap() + OrderCommission(); } } } if (totalProfit >= TakeProfit * g_pip * LotSize * 100000)
{
CloseAllOrders();
}
}
}
//+------------------------------------------------------------------+
//| Close all orders |
//+------------------------------------------------------------------+
void CloseAllOrders()
{
for (int i = OrdersTotal() - 1; i >= 0; i--)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderMagicNumber() == MagicNumber)
{
bool result = false;
if (OrderType() == OP_BUY)
{
result = OrderClose(OrderTicket(), OrderLots(), Bid, 3, White);
}
else if (OrderType() == OP_SELL)
{
result = OrderClose(OrderTicket(), OrderLots(), Ask, 3, White);
}
if (!result)
{
Print("Error closing order: ", GetLastError());
}
}
}
}
g_totalBuyOrders = 0;
g_totalSellOrders = 0;
Print("All grid orders closed");
}
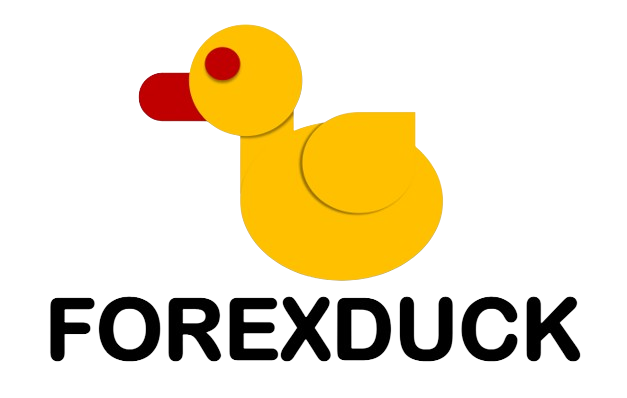
FOREXDUCK (นามปากกา) นักเขียนของเรามีประสบการณ์การเงินการลงทุนกว่า 10 ปี มีความเชี่ยวชาญในการวิเคราะห์ตลาด Forex และคริปโต โดยเฉพาะการวิเคราะห์ทางเทคนิค รวมถึงเทคนิคต่าง